Interrupts¶
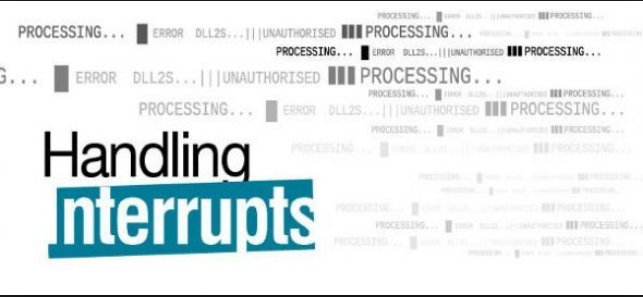
Interrupt Driven Low Power Pushbutton Activated LED (Version 1)¶
This example uses a MSP-EXP430G2 Board with a MSP430G2452 MCU in low power mode to pulse the red led when the user pushbutton is pressed. Current drain when not flashing the led is about 1 micro amp at 3V Vcc, and the binary executable created by Mecrisp-Across is 170 Bytes.
It also uses bit masks and absolute addresses when setting and resetting bits, such as the red led.
Dowload: pushbutton-interrupt.fs
\ Program Name: pushbutton-interrupt.fs
\ Date: 10 Nov 2018
\ Copyright 2018 t.porter <terry@tjporter.com.au>, licensed under the GPL
\ For Mecrisp-Across by Matthias Koch
\ http://mecrisp.sourceforge.net/
\
\ Features:
\ 1) Uses a MSP-EXP430G2 Board with a MSP430G2452 MCU
\ 2) Detect a S2 (p1.3) Push Button press using interrupts
\ 3) Pulses the red led once to indicate a Push Button (P1.3) press
\ 4) Uses Low Power Mode 4 until the Push Button is pressed, pulses the red led then goes back into LPM4.
\ Current measured at 3V while in LPM4 is ~1 micro amps
\ Binary size is 170 Bytes.
\ Note: It's important to keep the Interrupt Service Routines short and quick.
new \ new code to be cross compiled
+jtag \ activate JTAG mode
target \ Emulate the target ( We never leave the Host )
: read-pb ( -- ) \ manual diagnostic: read push button (at P1.3), 0 means button is held down.
P1IN @ binary . \ can only be used in target mode
decimal ;
: P1.3-interrupt-pending? \ manual diagnostic: 0 means a P1.3 Interrupt is NOT pending.
1 3 LSHIFT P1IFG cbit@ . ;\ can only be used in target mode
: init ( -- )
%11110111 P1DIR c! \ set P1.3 as input, the rest are outputs
%00001000 P1REN c! \ Enables pin's pull--up/down resistors
%00001000 P1IES c! \ P1.3 interrupt trigged H->L transition
%00001000 P1OUT c! \ set up only P1.3 with pull up resistor. If the pin's pull--up/down resistor is
; \ enabled, the corresponding bit in the PxOUT register selects pull-up or pull-down.
: red-off ( -- ) P1OUT c@ %00001000 AND P1OUT c! ; \ clr P1.6 without affecting the other bits
: red-on ( -- ) %01000000 P1OUT c@ OR P1OUT c! ; \ set P1.6 without affecting the other bits
: delay1 10000 0 DO LOOP ;
: delay ( delay -- ) 0 DO delay1 LOOP ;
: enable-p1.3-int P1IE c@ %00001000 OR P1IE c! ; \ enable P1.3 interrupt without affecting existing P1 values
: disable-p1.3-int P1IE c@ %11110111 AND P1IE c! ; \ disable P1.3 interrupt without affecting existing P1 values
: clear-p1.3-int P1IFG c@ %11110111 AND P1IFG c! ; \ clear p1.3-interrupt
: P1.3-int-handler ( -- ) \ P1.3 interrupt handler
wakeup \ wakeup the MCU from LPM4 mode
disable-p1.3-int \ disable the push button activated interrupt
clear-p1.3-int \ clear the push button interrupt flag
red-on \ turn on red led if PB pressed
25 delay \ This short 1 second delay will be ~25x longer in emulation (target mode)
red-off
enable-p1.3-int \ enable the push button activated interrupt
lpm4 \ go back into Low Power Mode 4
;
: main
init
clear-p1.3-int \ clear the push button interrupt flag
eint \ enable global interrupts
enable-p1.3-int \ enable the push button activated interrupt
lpm4 \ enter Low Power Mode 4 which stops almost everything for ~1uA current usage at 3V
;
host \ leave target mode, enter host mode
$FFE4 vector P1.3-int-handler \ push button interrupt vector
$FFFE vector main crosscompile flashtarget -jtag run \ crosscompile the code above and flash it to the target
\ hexdump \ print a neat hexdump of the cross compiled binary
\ t-listing \ t-listing shows what the cross compiler did
\ disimage \ disamige produces a disassembly listing of the target binary
hexdump
:10F800005742250037D2C2472500304157422300D6
:10F8100037F0F700C24723003041071208120912DF
:10F820000A125742250037F0F700C2472500B012F0
:10F830000CF8574221003840400008D7C248210048
:10F8400007433840190009433A4010271953099AD1
:10F85000FD2317530798F7235742210037F2C24779
:10F860002100B01200F832D0F8003A413941384155
:10F870003741B1C0F00002000013B240805A2001AD
:10F880003140800234406002F240F7002200F24230
:10F890002700F2422400F2422100B0120CF832D2CA
:10F8A000B01200F832D0F800FF3FFFFFFFFFFFFF6C
:10FFE000FFFFFFFF1AF8FFFFFFFFFFFFFFFFFFFF0D
:10FFF000FFFFFFFFFFFFFFFFFFFFFFFFFFFF7AF89D
:00000001FF
ok.
Interrupt Driven Low Power Pushbutton Activated LED (Version 2)¶
This version is identical to version 1 above but uses the Mecrisp time, space and error saving commands of bis! and bic!. The binary executable created by Mecrisp-Across is 132 Bytes, a 38 Byte saving over Version 1.
See Memory Mapped Word Lists for how this works and the extra files required.
Download: pushbutton-interrupt-1.fs
\ Program Name: pushbutton-interrupt-1.fs
\ Date: 10 Nov 2018
\ Copyright 2018 t.porter <terry@tjporter.com.au>, licensed under the GPL
\ For Mecrisp-Across by Matthias Koch
\ http://mecrisp.sourceforge.net/
\
\ Features:
\ 1) Uses a MSP-EXP430G2 Board with a MSP430G2452 MCU
\ 2) Detect a S2 (p1.3) Push Button press using interrupts
\ 3) Pulses the red led once to indicate a Push Button (P1.3) press
\ 4) Uses Low Power Mode 4 until the Push Button is pressed, pulses the red led then goes back into LPM4.
\ Current measured at 3V while in LPM4 is ~1 micro amps
\ Binary size is 132 Bytes.
\ Note: It's important to keep the Interrupt Service Routines short and quick.
new \ new code to be cross compiled
+jtag \ activate JTAG mode
target \ Emulate the target ( We never leave the Host )
: read-pb ( -- ) \ manual diagnostic: read push button (at P1.3), 0 means button is held down.
P1IN @ binary . \ can only be used in target mode
decimal ;
: P1.3-interrupt-pending? \ manual diagnostic: 0 means a P1.3 Interrupt is NOT pending.
1 3 LSHIFT P1IFG cbit@ . ;\ can only be used in target mode
: init ( -- )
%11110111 P1DIR c! \ set P1.3 as input, the rest are outputs
%00001000 P1REN c! \ Enables pin's pull--up/down resistors
%00001000 P1IES c! \ P1.3 interrupt trigged H->L transition
%00001000 P1OUT c! \ set up only P1.3 with pull up resistor. If the pin's pull--up/down resistor is
; \ enabled, the corresponding bit in the PxOUT register selects pull-up or pull-down.
: red-on ( P1OUT-P0-set ) %1 0 lshift P1OUT bis! ; \ set P1.6 without affecting the other bits
: red-off ( P1OUT-P0-clr ) %1 0 lshift P1OUT bic! ; \ clr P1.6 without affecting the other bits
: delay1 10000 0 DO LOOP ;
: delay ( delay -- ) 0 DO delay1 LOOP ;
: enable-p1.3-int ( P1IE-P3-set ) %1 3 lshift P1IE bis! ; \ enable P1.3 interrupt without affecting existing rest of register.
: disable-p1.3-int ( P1IE-P3-clr ) %1 3 lshift P1IE bic! ; \ disable P1.3 interrupt without affecting existing rest of register.
: clear-p1.3-int ( P1IFG-P3-clr ) %1 3 lshift P1IFG bic! ; \ clear p1.3-interrupt without affecting existing rest of register.
: P1.3-int-handler ( -- ) \ P1.3 interrupt handler
wakeup \ wakeup the MCU from LPM4 mode
disable-p1.3-int \ disable the push button activated interrupt
clear-p1.3-int \ clear the push button interrupt flag
red-on \ turn on Red led if PB pressed
25 delay \ This short 1 second delay will be ~25x longer in emulation (target mode)
red-off
enable-p1.3-int \ enable the push button activated interrupt
lpm4 \ go back into Low Power Mode 4
;
: main
init
clear-p1.3-int \ clear the push button interrupt flag
eint \ enable global interrupts
enable-p1.3-int \ enable the push button activated interrupt
lpm4 \ enter Low Power Mode 4 which stops almost everything for ~1uA current usage at 3V
;
host \ leave target mode, enter host mode
$FFE4 vector P1.3-int-handler \ push button interrupt vector
$FFFE vector main crosscompile flashtarget -jtag run \ crosscompile the code above and flash it to the target
hexdump \ print a neat hexdump of the cross compiled binary
\ t-listing \ t-listing shows what the cross compiler did
\ disimage \ disamige produces a disassembly listing of the target binary